Form input validation is a critical aspect of web development. It ensures that users enter the necessary and correctly formatted information before it’s processed. This blog post will delve into form validation using HTML5 and JavaScript, providing practical code examples.
Why Validate Forms?
Validation is essential for:
- Ensuring data integrity
- Enhancing user experience
- Preventing security vulnerabilities
Web Designer Career Path
Our Web Designer Career Path training series is thoughtfully curated to cater to this growing need, offering an all-encompassing educational journey for those aspiring to thrive in the realm of web design. This series offers an in-depth exploration of both the theoretical foundations and practical applications of web design, ensuring participants are fully equipped to craft engaging and functional websites.
Basic HTML5 Form Validation
HTML5 introduced built-in form validation mechanisms that are easy to implement. Here’s a simple example:
<form>
<label for="email">Email:</label>
<input type="email" id="email" required>
<button type="submit">Submit</button>
</form>
The required
attribute in the input tag ensures that the user cannot submit the form without filling in this field.
JavaScript Check Input Type
To take validation further, JavaScript allows for more complex checks:
document.getElementById('email').addEventListener('input', function (event) {
if (this.validity.typeMismatch) {
this.setCustomValidity('Please enter a valid email address.');
} else {
this.setCustomValidity('');
}
});
This code snippet adds an event listener that checks the type of the input. If it’s not an email, it displays a custom validation message.
Advanced Form Validation Techniques
While HTML5 validation is useful, it’s often necessary to implement more advanced validation logic with JavaScript.
Checking Input on the Fly
document.getElementById('username').addEventListener('input', function (event) {
var pattern = /^[a-zA-Z0-9]*$/;
if (!pattern.test(this.value)) {
this.setCustomValidity('Username must contain only letters and numbers.');
} else {
this.setCustomValidity('');
}
});
Form Validation on Submission
document.getElementById('myForm').addEventListener('submit', function (event) {
// Check if all fields are valid
if (!this.checkValidity()) {
event.preventDefault();
// Optionally, provide feedback
}
});
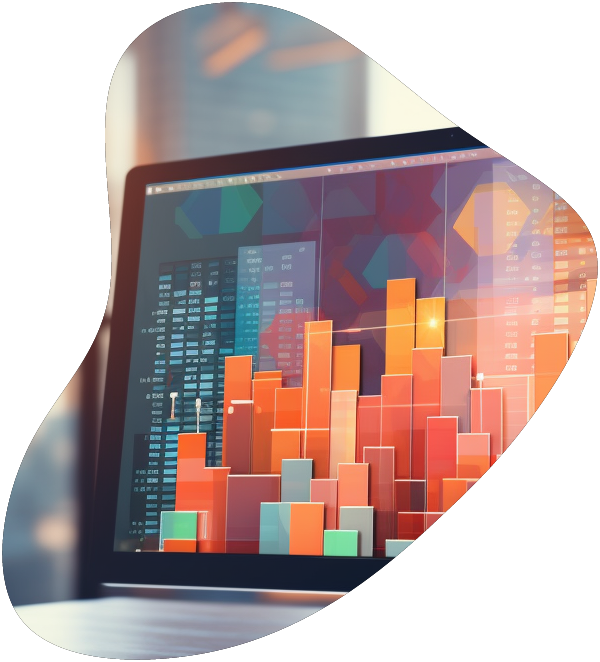
Lock In Our Lowest Price Ever For Only $16.99 Monthly Access
Your career in information technology last for years. Technology changes rapidly. An ITU Online IT Training subscription offers you flexible and affordable IT training. With our IT training at your fingertips, your career opportunities are never ending as you grow your skills.
Plus, start today and get 30 days for only $1.00 with no obligation. Cancel anytime.
Using HTML5 Constraints for Input Validation
HTML5 offers several attributes to enforce different constraints, such as pattern
, min
, max
, and maxlength
.
Example: Using Pattern for Regex Validation
<input type="text" id="zipcode" pattern="^\d{5}(-\d{4})?$" title="Enter a valid US zipcode">
Form Validation Feedback
Providing immediate feedback to the user is crucial for a good experience. Here’s how you can display validation errors next to the input fields:
document.getElementById('email').addEventListener('invalid', function (event) {
event.preventDefault();
// Display an error message
var errorMessage = document.getElementById('email-error');
errorMessage.textContent = 'Please enter a valid email address.';
});
Conclusion
Combining HTML5 and JavaScript for form validation provides robust solutions for front-end form validation. By ensuring that users input data correctly, developers can maintain data integrity, improve user experience, and secure their applications against invalid data.
Key Term Knowledge Base: Key Terms Related to Understanding Form Input Validation in HTML5 and JavaScript
Form input validation is a critical aspect of web development that ensures the data collected through web forms is correct, secure, and useful. It involves checking the data entered by users against a set of rules or constraints before it is processed or stored. This process helps in preventing incorrect or malicious data from entering the system, enhancing the overall user experience, and securing the web application from potential vulnerabilities. Knowing the key terms related to form input validation in HTML5 and JavaScript is essential for developers to efficiently implement robust validation mechanisms in their web applications.
Term | Definition |
---|---|
HTML5 Validation | The process of ensuring the data entered into form fields meets the standards set by HTML5 attributes without needing to use JavaScript for basic validation. |
JavaScript Validation | The method of checking the correctness of the data on the client side using JavaScript, allowing for more dynamic and customized validation logic. |
required Attribute | An HTML5 attribute used to specify that a field must be filled out before submitting the form. |
pattern Attribute | An HTML5 attribute that specifies a regular expression against which the input’s value is checked, for enforcing specific formatting. |
type Attribute | An HTML5 attribute that defines the type of data expected in an input field, such as email , number , or date , providing built-in validation for the specified type. |
min and max Attributes | HTML5 attributes used to define the minimum and maximum values for input fields of type number , range , date , etc. |
maxlength and minlength Attributes | HTML5 attributes that define the maximum and minimum lengths of text that can be entered in input fields like text and password . |
novalidate Attribute | An attribute that can be added to a <form> tag to disable HTML5 validation, allowing the developer to handle validation purely with JavaScript. |
Regular Expression (Regex) | A sequence of characters that forms a search pattern, often used for string matching and validation in programming, including form input validation. |
Client-side Validation | Validation performed in the user’s browser before the data is submitted to the server, improving user experience by providing immediate feedback. |
Server-side Validation | Validation performed on the server after the data has been submitted, providing a secure layer of validation that cannot be bypassed by the client. |
Form Validation API | A collection of properties and methods provided by HTML5 and JavaScript for validating form input, including custom validation messages and validity state checks. |
setCustomValidity() Method | A JavaScript method used to set a custom error message for an input element when the built-in validation rules do not suffice. |
checkValidity() Method | A JavaScript method used to check whether an input element’s value satisfies its constraints, returning true or false . |
Constraint Validation API | A set of JavaScript APIs that allow for more detailed control over the validation of form fields, including checking validity and setting custom validation messages. |
validity Property | A property of input elements that contains several boolean properties related to the validity of the element’s value (e.g., patternMismatch , valueMissing ). |
addEventListener() Method | A JavaScript method used to attach an event listener to an element, commonly used to listen for form submission events and perform validation. |
preventDefault() Method | A JavaScript method used within an event handler to stop the default action of an element from happening, often used to prevent a form from submitting when validation fails. |
Asynchronous Validation | The process of validating form input against a server or external API, requiring asynchronous JavaScript code, often used for checking uniqueness (e.g., username availability). |
Input Sanitization | The process of cleaning and preparing user input data, typically by removing potentially harmful or unwanted characters, to prevent security vulnerabilities like SQL injection. |
Cross-site Scripting (XSS) Prevention | A security measure in form validation that involves sanitizing user input to prevent the execution of malicious scripts on the client side. |
Understanding these terms and their applications is fundamental for developers aiming to create secure, user-friendly web applications with effective input validation mechanisms.
Frequently Asked Questions Related to Form Validation With HTML5 and Javascript
What is form input validation, and why is it necessary?
Form input validation is the process of ensuring that the data supplied by a user in a form meets specific criteria before it is accepted. This is necessary to maintain data integrity, provide a good user experience, and prevent security vulnerabilities. For example, validating an email address ensures that the data corresponds to the format of a valid email, which is crucial for follow-up communications.
How does HTML5 help in form input validation?
HTML5 simplifies form input validation by providing built-in attributes that automatically check the data entered by the user against certain rules. Attributes such as type, required, pattern, min, max, and step can be used to specify the kind of data expected in an input field. When a form is submitted, the browser checks all the input fields with these attributes and prevents the form from being submitted if any data does not comply.
Can form input validation be bypassed?
While client-side form input validation provides an initial layer of checking and improves user experience, it can be bypassed by users with technical knowledge who can modify the client-side code. Therefore, it is crucial to also implement server-side validation to ensure that the data adheres to the necessary criteria before it is processed or stored, offering a more secure validation mechanism.
What are the common types of data validated in forms?
Common types of data that are validated in forms include personal information like names and addresses, user credentials like usernames and passwords, communication details like email addresses and phone numbers, and financial information like credit card numbers. Each type of data has specific formats and criteria that need to be met, such as length, characters allowed, and the format of the entry (like an email address having an “@” symbol and a domain).
How can I provide user feedback if their input is invalid?
Providing user feedback for invalid input can be done by utilizing the built-in browser tooltip that appears when the data entered does not comply with the validation rules. For a more customized approach, JavaScript can be used to display error messages next to the relevant input field or in a designated area of the form. This feedback should be clear and informative, guiding the user to correct the input and explaining why the entered data is not acceptable.